Entry #5 - Fuh-Yeh! Transform!
- jamescgonzales
- Jan 28, 2021
- 2 min read
Updated: Jan 30, 2021
Image Enhancement Part3 - Fourier Transform
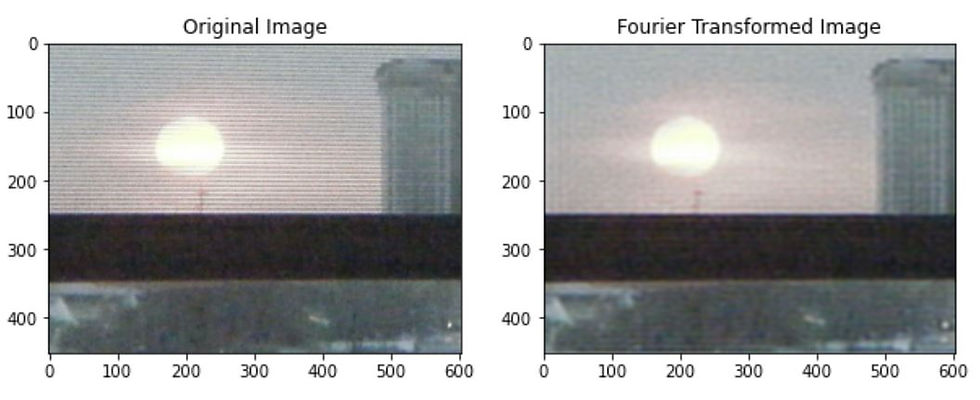
Way back in 2014, while waiting to board my plane to Davao. I took a photo of the sunset. It was a crappy shot. For some reason, it stayed on my storage drive. Never do I know that I will make use of it, until now.
Images can be represented as a superposition of waves or wave patterns. And some images have some periodic noise in them- like the mentioned photo. Fortunately, by doing Fourier transform on the image, these periodic patterns can be further observed and masked, enhancing the image.
So let's enhance the image with periodic noise using the Fourier transform.
But first, let's load the required libraries:
import numpy as np
import matplotlib.pyplot as plt
from skimage.io import imread, imshow
from skimage.color import rgb2gray
Now let's load the image and convert it to grayscale to simplify the process.
Using numpy, we can get the fft of the image using np.fft.fft2. To center the signal, we need to user np.fft.fftshift.
fft_img = np.fft.fft2(img_g)
fft_centered = np.fft.fftshift(fft_img)
Below is the Centered Spectrum.
The Lines and the bright specks above describes the periodic waveform of the image. Notice the bright centered speck. That is the DC component of the image, which contains information on the image's intensity values. We can mask these periodic noises, for this example, the vertical white lines.
To mask the white line, we can get the line's coordinates and replace it with a small value, for this example we will be using 1.
Now, let's transform the modified Fourier to the spatial domain.
Looks like the lines on the image are minimized but some parts of the window bar seems to be blurred.
To improve the image, we can still use low pass filter function below. Instead of the masked fft_centered2.
def distance(point1, point2):
return np.sqrt((point1[0]-point2[0])**2 + (point1[1]-point2[1])**2)
def lp_filter(d0, imgShape):
base = np.zeros(imgShape[:2])
rows, cols = imgShape[:2]
fft_centered = (rows/2,cols/2)
for x in range(cols):
for y in range(rows):
if distance((y,x),fft_centered) < d0:
base[y,x] = 1
return base
lowpass_center = fft_centered * lp_filter(50, img_g.shape)
LowPass = np.fft.ifftshift(lowpass_center)
inverse_LowPass = np.fft.ifft2(LowPass)
Looks better. Now let's process the image in its RGB channels.
def rgb_transform(img, channel):
rgb_fft = np.fft.fftshift(np.fft.fft2(img[:, :, channel]))
LowPassCenter = rgb_fft * lp_filter(50, img_g.shape)
LowPass = np.fft.ifftshift(LowPassCenter)
inverse_LowPass = np.fft.ifft2(rgb_fft)
inverse_LowPass = np.fft.ifft2(LowPassCenter)
return abs(inverse_LowPass).clip(0, 255)
fig, ax = plt.subplots(2, 1, figsize=(10,10))
new_image = np.dstack([red_channel.astype(int),
green_channel.astype(int),
blue_channel.astype(int)])
ax[0].imshow(img)
ax[0].set_title('Original Image');
ax[1].imshow(new_image);
ax[1].set_title('Fourier Transformed Image');
And that's it! The image started to be crappy and I believe I made it a little less of a crap by using Fourier Transformation and Filtering Method. :)
In summary, we now know that the image is like a signal that can be represented by wave or wave patterns. Fourier transformation transforms the image presentation from spatial to the frequency domain, in which we can observe and modify the image. The periodic noise, represented by the specs and lines in the frequency domain, can be masked by a small value to enhance the image. It is important to avoid modifying the DC component of the image since it contains the image's intensity values. And finally, the use of a filter can help improve the image by minimizing the impact on other parts of the image.
Comments