Template Matching
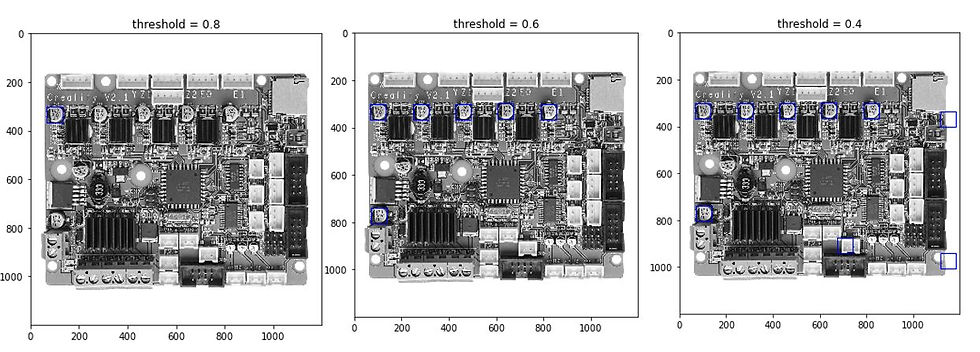
Template matching is a matching technique to identify the occurrence of an image patch from a given image. This is practically useful in image detection and object tracking. As a use case, it can help detect anomalies in circuit board components during its manufacturing process.
Let's get started by loading our libraries.
from skimage.io import imread, imshow, imsave
from skimage.color import rgb2gray
from skimage.feature import match_template
from skimage.feature import peak_local_max
import numpy as np
import matplotlib.pyplot as plt
Here, we have a new function from scikit-image - match_template. This uses fast, normalized cross-correlation to find instances of the template in the image.
Let's load our image. We will use the image from a 3D printer's circuit board (Creality 3D CR-10S 12V Mainboard V2.1 PCB), which is sold at Aliexpress https://www.aliexpress.com/item/4000414212580.html. The image contains its components, and our goal is to prepare a template and find matching components within the board.
Template matching can work in RGB, but to simplify our example, our template is also somewhat grayscale will use the Grayscale version of the image. Let's get the grayscale image.
Now, for the template. We will select an object within the image by getting the pixel coordinates. For this example, I set the 100uf 35V Aluminum Capacitor. I once again used MS paint to get the pixel coordinate information.
We will now match the template in the main image using the match_template function, as shown below.
Observing the result above are the bright specks. We can assume that these are the matched objects in the image. We can also assume that since the template was taken from this image, the maximum value should be the template's coordinates.
Bounded with the blue box is the template we used.
To get the multiple matches of the template to the image, we can adjust the threshold of the template's correlation to the image.
Using a function, we can loop through the given thresholds.
def plot_match(img_gray, thresh, templ):
figure = plt.figure(figsize=(5, 5))
imshow(board_gray)
template_width, template_height = templ.shape
result = match_template(img_gray, templ)
for x, y in peak_local_max(result, threshold_abs=thresh):
rect = plt.Rectangle((y, x), template_height, template_width,
color='b', fc='none')
plt.gca().add_patch(rect);
thr = [0.4, 0.6, 0.8]
for x, y in enumerate(thr):
plot_match(board_gray, thr[x], template )
plt.title(f'threshold = '+str(y))
What if we resize the template? Will it still match something from the image?
thr = [0.4, 0.6, 0.8]
for x, y in enumerate(thr):
plot_match(board_gray, thr[x], template_resized)
plt.title(f'threshold = '+str(y))
It didn't match any target object.
What about if we rotate the template?
Also couldn't match the target object.
What if we change the contrast?
thr = [0.4, 0.6, 0.8]
for x, y in enumerate(thr):
plot_match(board_gray, thr[x], template_contrast)
plt.title(f'threshold = '+str(y))
Like rescaling and rotation, changing the contrast will fail the matching or template to the image.
In summary:
Increasing the threshold close to 1, would get the perfect match, which is the template itself. On the other hand, reducing it too low would capture objects which are not close to the template. For the image above, we can have 0.6 to capture the 6 capacitors, which are similar to the template.
By increasing the template's size, the template matching would not detect the target object since the template is just being used to scan through the image to find a match given its characteristics. Therefore we can say that the template matching is scale invariant.
Rotating the size would also fail to match or detect the target object unless there is also a rotated object similar to the modified template. Therefore we can say that the template is rotationally invariant.
Intuitively, changing the contrast of the image would cause the template matching to detect the target object for the same reason as scaling and rotational invariant. We can say that that template matching is also intensity invariant.
Image Reference:
PCB Board image from : https://www.aliexpress.com/item/4000414212580.html
Comments